Learn Python
Python Introduction |
Python Installation |
Python Hello World |
Python Tokens |
Python Program Structure |
Python Variables and Assignments |
Python Input Output |
Python Data Types |
Python Mutable Immutable |
Python Opeartors |
Python Expression |
Python if statement |
Python range method |
Python Loop |
Python break continue |
Python Loop else |
Python Nested Loop |
Python Data Types
Data type of a variable tell us about the data contained by variable and allowed associated operations on it.
Python Data Types
Data type of a variable tell us about the data contained by variable and allowed associated operations on it.
Python has following built-in data types:
Numbers (int, float, complex, Boolean),
None,
Sequences (String, List, and Tuples) and
Mapping (Dictionary)
a) int (Integer) – Integer represents whole numbers without any fractional part. There are two types of Integers in Python –
i. Integer (signed) – Whole Number, with unlimited size, depends upon computer memory. Python has single data type (int) for any integer, whether big / long or small.
Example : x = 20
ii. Booleans – These represent truth values False and True. Boolean values are plain integers where False means 0 and True means 1. bool(0) return False, bool(1) returns True.
Example : x = True
b) float (floating point numbers) – Represent real number or floating point number, i.e. numbers with fractional part. A Floating point number in Python can have maximum 53 bits of precision.
Note: 25 is an integer while 25.0 is a floating point number.
Example: p = 25.908787889
c) Complex Numbers: Complex numbers in Python are made up of pairs of real and imaginary numbers. Python represents complex number in the form A + Bj, where A is the real part and B is the imaginary part. J or j is the imaginary number, equal to the square root of -1 i.e. .
A and B both are internally represented as floating point number.
Python displays complex numbers in parentheses when they have a non-zero real part.
Complex numbers are a composite quantity made of two parts: the real part and imaginary part, both of which are represented internally as float values.
These two parts of complex number can be accessed through attributes – real & imag.
complexNumber.real - gives the real part as a float
complexNumber.imag - gives the imaginary part as a float
d) String (str) : A string data type allows us to hold string data i.e. any number of valid characters into a set of quotation marks. In Python 3.x string store Unicode characters.
Example :
‘125AnjeevSingh’ , “$%@#87Singh Academy”, “??*[],.<>”
In Python, String is a sequence of characters and each character can be individually accessed using its index position.
There are two types of indexing in Python -
Backward Indexing 0 1 2 3 4 5
A N J E E V
Forward Indexing -6 -5 -4 -3 -2 -1
e) List - is a list of comma separated values of any data type between square brackets. It can be changed. E.g.
p = [1, 2, 3, 4] m = [‘a’, ‘e’, ‘i’, ‘o’, ‘u’]
q = [“Anjeev”, “kumar”, “singh”]
r = [“Mohit”, 102, 85.2, True]
f) Tuple - is a list of comma separated values of any data type between parentheses. It cannot be changed. Eg.
p = (1, 2, 3, 4) m = (‘a’, ‘e’, ‘i’, ‘o’, ‘u’)
q = (“Anjeev”, “kumar”, “singh”)
r = (“Mohit”, 102, 85.2, ‘M’, True)
g) Dictionary – is an unordered set of comma-separated key : value pairs within curly braces.
rec = {‘name’:“Mohit”, ‘roll’: 102, ‘marks’ : 85.2, ‘sex’: ‘M’, ‘ip’: True}
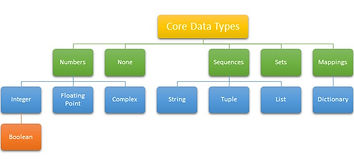